在上一篇文章中介绍了如何使用钱包来发送btc交易,本文将介绍一些区块链的基础命令。
block命令主入口
block
命令目前提供以下几个子命令:
chaininfo
:查看区块链的基本信息,包括链名称、区块高度、出块难度等。getblock
:查看区块信息,包括区块哈希、区块高度、区块时间戳、区块父哈希、区块交易数量等。getcount
:查看区块高度。gethash
:查看区块哈希。getheader
:查看区块头信息。
// blockchainCmd 创建一个与区块链相关的命令
func (c *WalletCommand) blockchainCmd() *cobra.Command {
cmd := &cobra.Command{
Use: "block",
Short: "Blockchain related commands",
Long: "Blockchain related commands",
}
cmd.AddCommand(
c.chainInfoCmd(),
c.blockCountCmd(),
c.blockHashCmd(),
c.blockHeaderCmd(),
c.blockCmd(),
)
return cmd
}
// blockCountCmd 创建一个获取当前区块数量的命令
func (c *WalletCommand) blockCountCmd() *cobra.Command {
return &cobra.Command{
Use: "getcount",
Short: "Get the current block count",
Long: "Get the current block count, example: ./wallet block getcount",
RunE: func(cmd *cobra.Command, args []string) error {
bc, err := client.GetBlockCount()
if err != nil {
return errors.Wrap(err, "failed to get block count")
}
fmt.Printf("block count: %d\n", bc)
return nil
},
}
}
// blockHashCmd 创建一个通过区块编号获取区块哈希的命令
func (c *WalletCommand) blockHashCmd() *cobra.Command {
return &cobra.Command{
Use: "gethash",
Short: "Get the block hash by block number",
Long: "Get the block hash by block number, example: ./wallet block gethash 100",
RunE: func(cmd *cobra.Command, args []string) error {
bc, err := strconv.ParseInt(args[0], 10, 64)
if err != nil {
return errors.Wrap(err, "failed to parse block number")
}
hash, err := client.GetBlockHash(bc)
if err != nil {
return errors.Wrap(err, "failed to get block hash")
}
fmt.Printf("block hash: %s\n", hash.String())
return nil
},
}
}
// blockHeaderCmd 创建一个通过区块哈希获取区块头的命令
func (c *WalletCommand) blockHeaderCmd() *cobra.Command {
return &cobra.Command{
Use: "getheader",
Short: "Get the block header by block hash",
Long: "Get the block header by block hash, example: ./wallet block getheader hash",
RunE: func(cmd *cobra.Command, args []string) error {
hash, err := chainhash.NewHashFromStr(args[0])
if err != nil {
return errors.Wrap(err, "failed to parse block hash")
}
header, err := client.GetBlockHeader(hash)
if err != nil {
return errors.Wrap(err, "failed to get block header")
}
fmt.Println("block header:")
fmt.Printf("\t version: %d\n", header.Version)
fmt.Printf("\t prev block: %s\n", header.PrevBlock.String())
fmt.Printf("\t merkle root: %s\n", header.MerkleRoot.String())
fmt.Println("\t timestamp: ", header.Timestamp)
fmt.Printf("\t bits: %d\n", header.Bits)
fmt.Printf("\t nonce: %d\n", header.Nonce)
return nil
},
}
}
// blockCmd 创建一个通过区块哈希获取区块的命令
func (c *WalletCommand) blockCmd() *cobra.Command {
return &cobra.Command{
Use: "getblock",
Short: "Get the block by block hash",
Long: "Get the block by block hash, example: ./wallet block getblock hash",
RunE: func(cmd *cobra.Command, args []string) error {
hash, err := chainhash.NewHashFromStr(args[0])
if err != nil {
return errors.Wrap(err, "failed to parse block hash")
}
block, err := client.GetBlock(hash)
if err != nil {
return errors.Wrap(err, "failed to get block")
}
fmt.Printf("block: %s has %d transactions\n", args[0],len(block.Transactions))
return nil
},
}
}
// chainInfoCmd 创建一个获取链信息的命令
func (c *WalletCommand) chainInfoCmd() *cobra.Command {
return &cobra.Command{
Use: "chaininfo",
Short: "Get the chain information",
Long: "Get the chain information, example: ./wallet block chaininfo",
RunE: func(cmd *cobra.Command, args []string) error {
info, err := client.GetBlockChainInfo()
if err != nil {
return errors.Wrap(err, "failed to get chain info")
}
fmt.Println("chain info:")
fmt.Printf("\t chain: %s\n", info.Chain)
fmt.Printf("\t blocks: %d\n", info.Blocks)
fmt.Printf("\t headers: %d\n", info.Headers)
fmt.Printf("\t best block hash: %s\n", info.BestBlockHash)
fmt.Printf("\t difficulty: %f\n", info.Difficulty)
return nil
},
}
}
操作示例
# 查看block命令信息
$ ./btc_wallet block
Blockchain related commands
Usage:
wallet block [command]
Available Commands:
chaininfo Get the chain information
getblock Get the block by block hash
getcount Get the current block count
gethash Get the block hash by block number
getheader Get the block header by block hash
Flags:
-h, --help help for block
Use "wallet block [command] --help" for more information about a command.
# 查看区块链基本信息
$ ./btc_wallet block chaininfo
chain info:
chain: testnet3
blocks: 2902433
headers: 2902433
best block hash: 00000000eaeedf7f4d87ae7221cbe899be749ba24737cb633c846e0695927880
difficulty: 1.000000
# 查看区块高度
$ ./btc_wallet block getcount
block count: 2902433
# 查看区块哈希
$ ./btc_wallet block gethash 2902433
block hash: 00000000eaeedf7f4d87ae7221cbe899be749ba24737cb633c846e0695927880
# 查看区块信息,目前仅展示给区块包含了多少笔交易
$ ./btc_wallet block getblock 00000000eaeedf7f4d87ae7221cbe899be749ba24737cb633c846e0695927880
block: 00000000eaeedf7f4d87ae7221cbe899be749ba24737cb633c846e0695927880 has 3611 transactions
# 查看区块头信息
$ ./btc_wallet block getheader 00000000eaeedf7f4d87ae7221cbe899be749ba24737cb633c846e0695927880
block header:
version: 536870912
prev block: 000000000000003921c892019c31d941f26153214064ea876cba76f0385cb3bf
merkle root: f821be766519b8de1eea39195cf1318eb5ed62f95c203aa0497ae04af66238d4
timestamp: 2024-09-02 22:39:04 +0800 CST
bits: 486604799
nonce: 1329847566
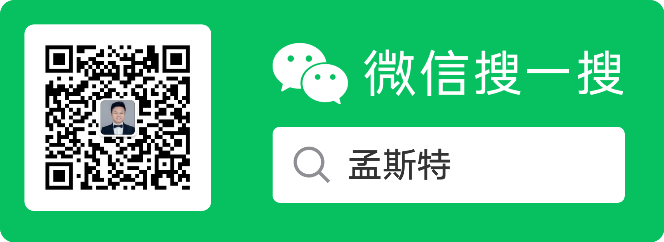
声明:本作品采用署名-非商业性使用-相同方式共享 4.0 国际 (CC BY-NC-SA 4.0)进行许可,使用时请注明出处。
Author: mengbin
blog: mengbin
Github: mengbin92
cnblogs: 恋水无意
腾讯云开发者社区:孟斯特